class: bottom, left, title-slide # Working with Files Using
fs
### Garrick Aden-Buie ### September 24, 2018 --- class: center middle inverse # fs ## File System Operations -- ### (Work with *paths*, *files*, and *directories* within R) -- ### <pre><code>install.packages("fs")</code></pre> --- ## Overview of Functions in `fs` <style type="text/css"> .map-image { text-align: center; width: 100%; } div.map-image p img { max-height: 475px; } </style> <div style="font-size: 1.75em"> <table class='plain'> <thead> <tr> <th style="text-align:left;"> Prefix </th> <th style="text-align:left;"> Functionality </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> <code>path_</code> </td> <td style="text-align:left;"> Create, find, expand, work with paths </td> </tr> <tr> <td style="text-align:left;"> <code>file_</code> </td> <td style="text-align:left;"> Create, delete, copy, move, show, check files </td> </tr> <tr> <td style="text-align:left;"> <code>dir_</code> </td> <td style="text-align:left;"> Create, delete, list, check directories </td> </tr> </tbody> </table> </div> --- class: center middle inverse ## What are _paths_, _files_ and _directories_? -- ### Maps as a metaphor for the file system --- layout: true ## Directories --- United States .map-image[ 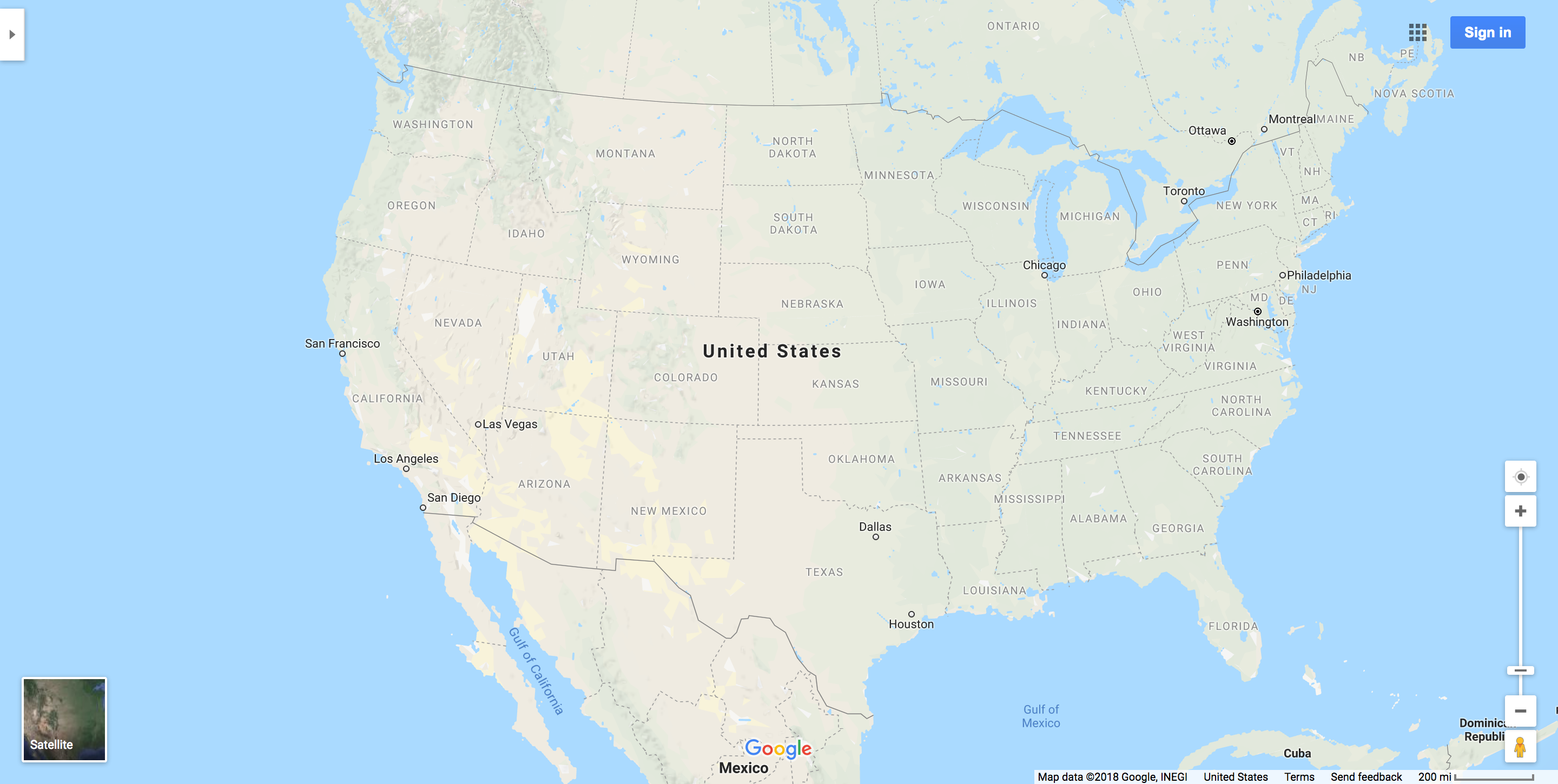 ] --- United States > Florida .map-image[ 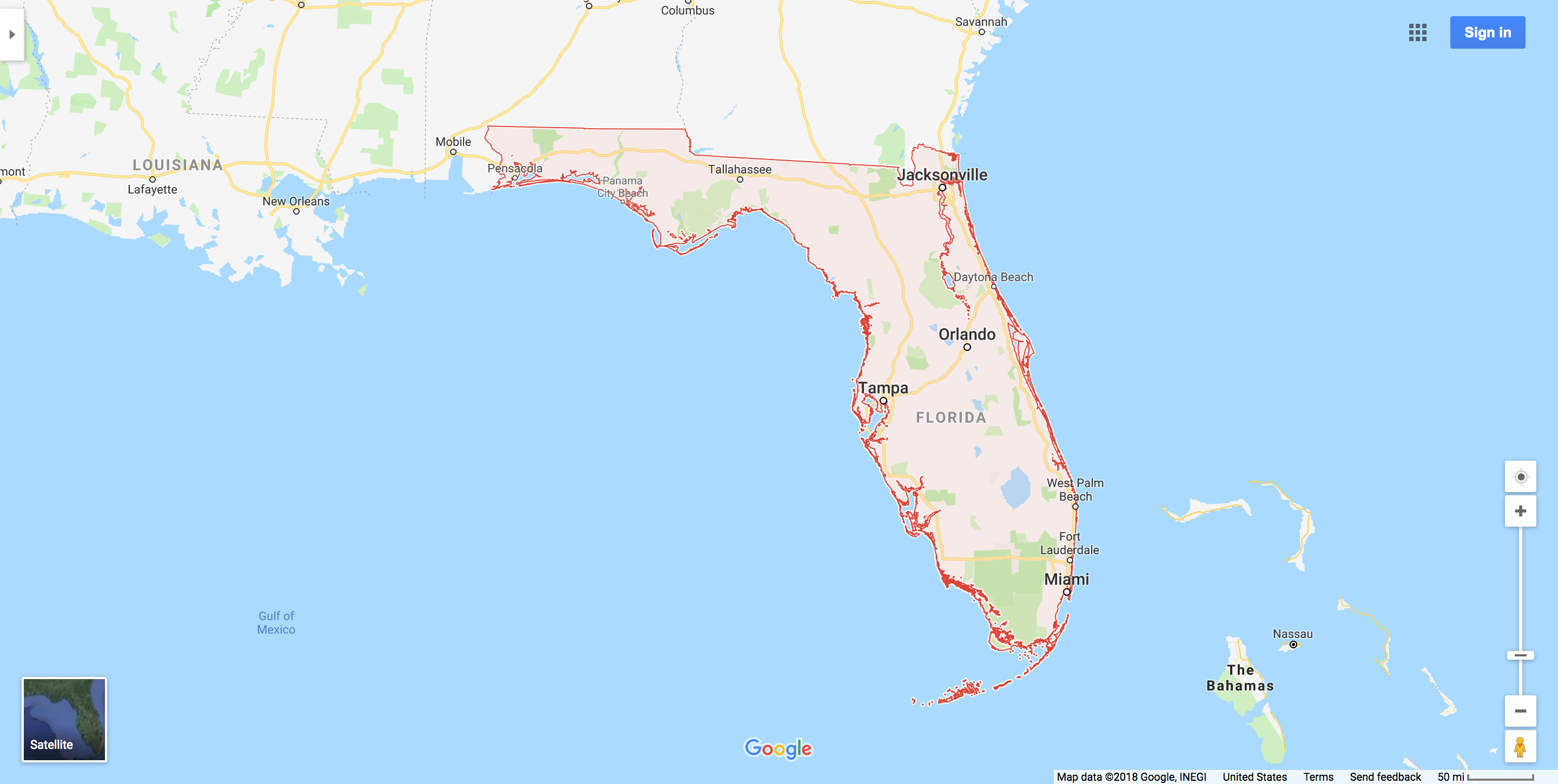 ] --- United States > Florida > 33704 .map-image[ 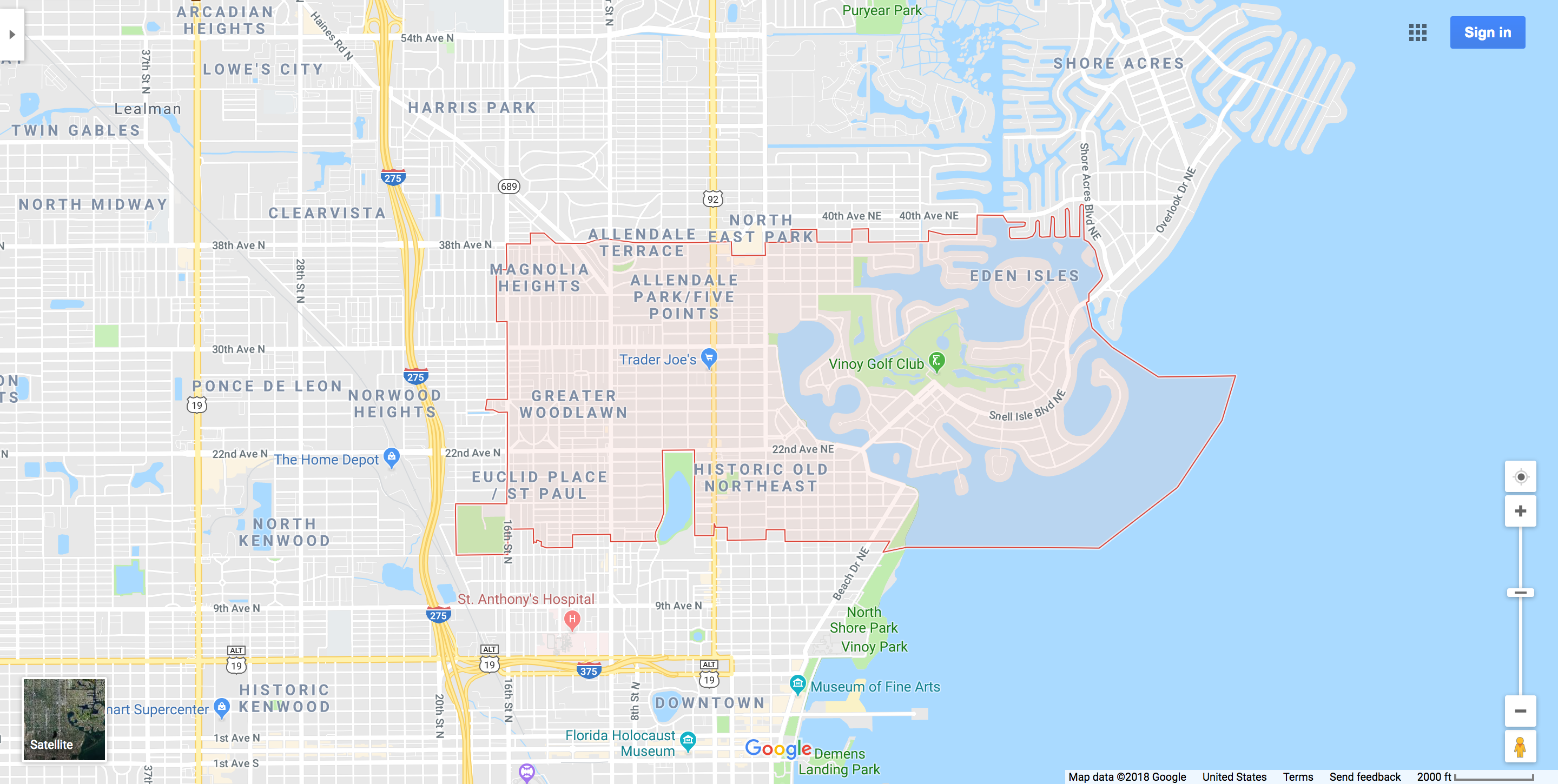 ] --- layout: false ## Path 720 35th Ave N., St. Petersburg, FL, 33704 .map-image[ 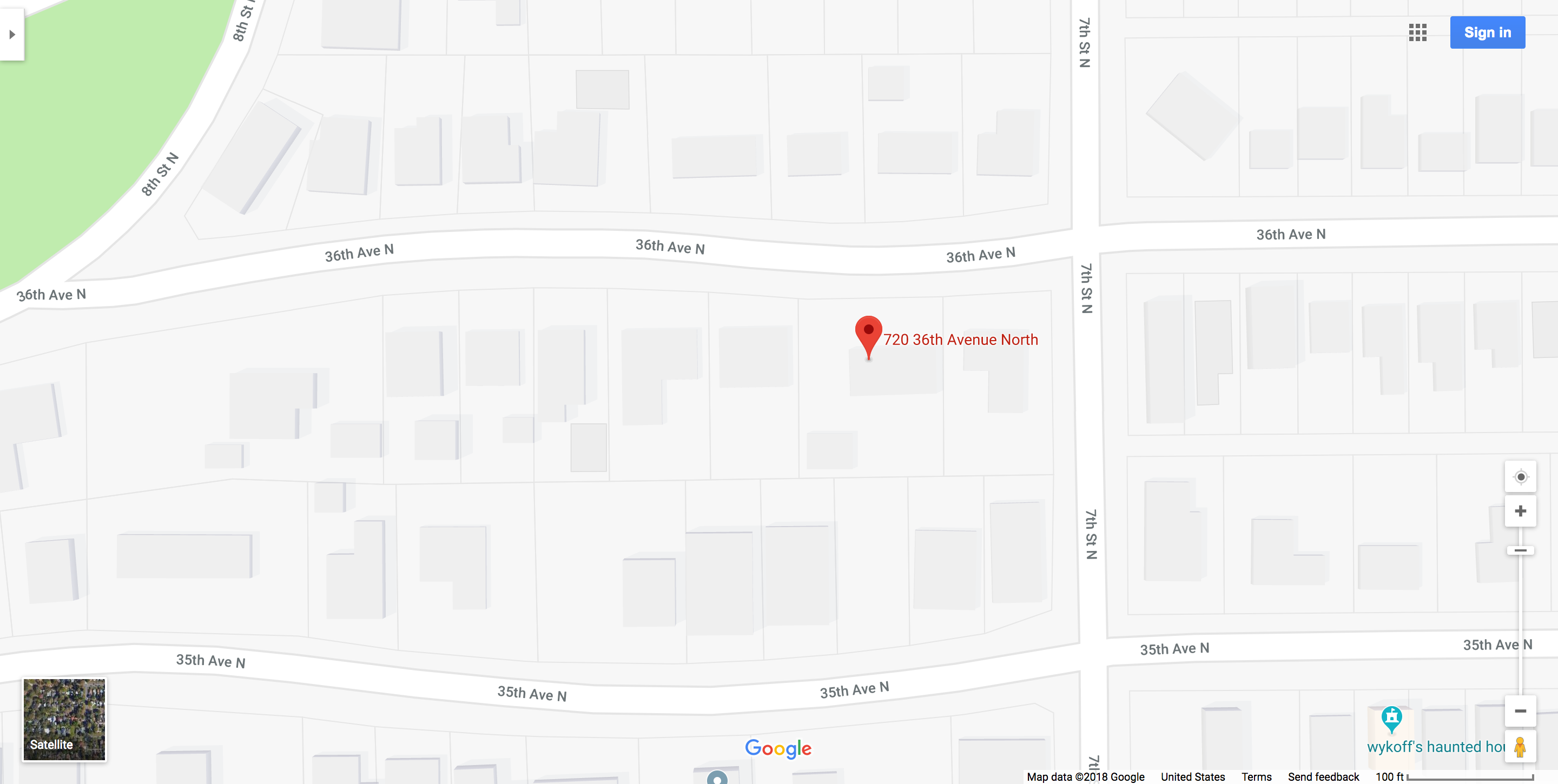 ] --- layout: true ## File --- The actual thing that is at the path... .map-image[ 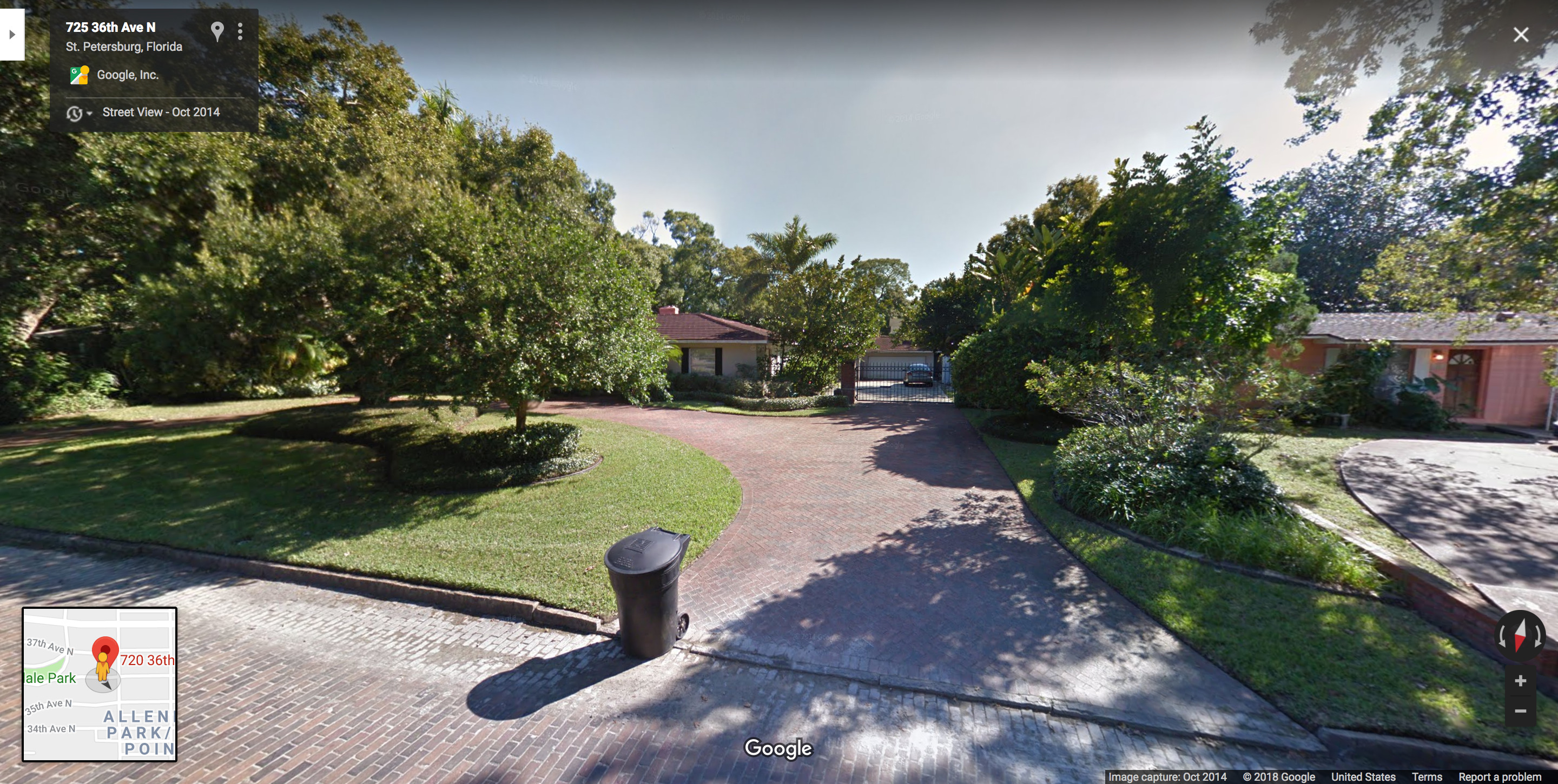 ] --- ...that may or may not actually be there .map-image[ 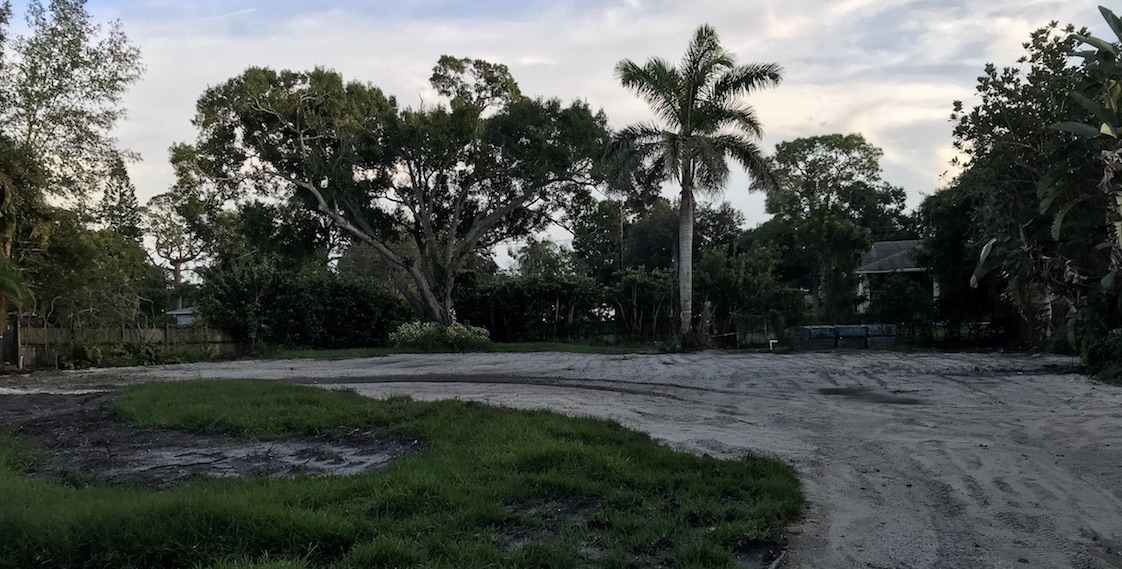 ] --- layout: false ## File Paths are Platform Dependent These all point to the same file, in different places. (Windows, Mac, Web, Network) .left-column[ 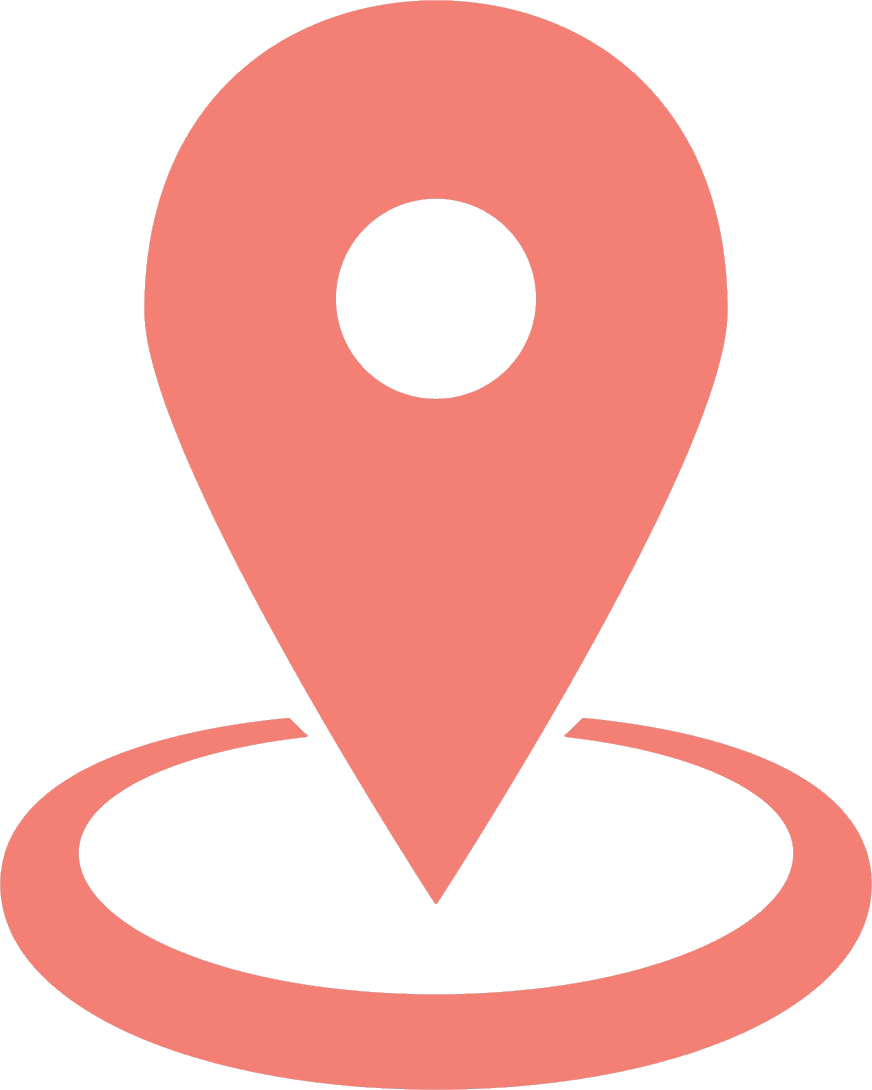 ] .right-column[ .line-height-1-5[`C:\Libraries\Documents\Documents\images\my-photo.jpg`] .line-height-1-5[`/User/username/Documents/images/my-photo.jpg`] .line-height-1-5[`https://username.com/images/my-photo.jpg`] .line-height-1-5[`\\net.edu\Users\username\Documents\images\my-photo.jpg`] ] ??? A selection of file paths pointing to the same file on several different systems: - Windows - Mac - Web - Network --- ## Relative Paths Compared with **absolute** paths, **relative** paths assume the starting point. .left-column[ 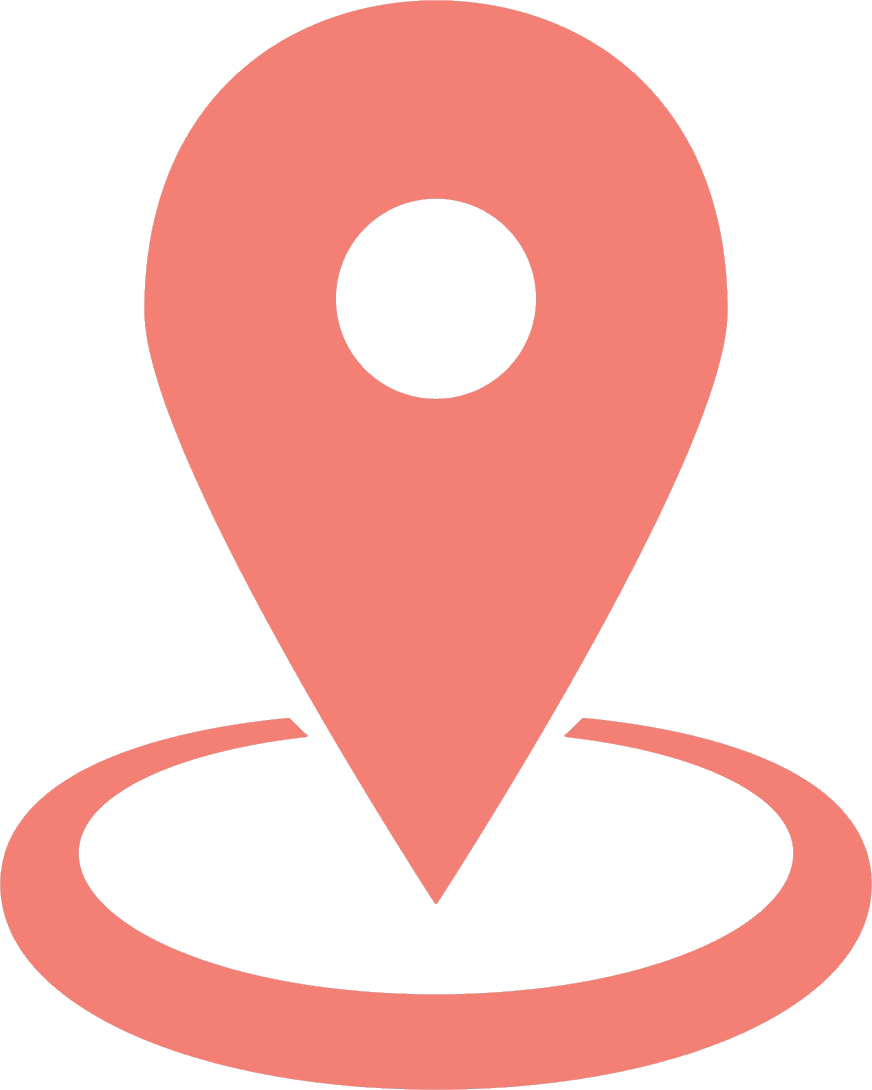 ] .right-column[ .line-height-1-5[`.\images\my-photo.jpg`] .line-height-1-5[`../../images/my-photo.jpg`] .line-height-1-5[`images/my-photo.jpg`] .line-height-1-5[`~\Documents\papers\\..\images\my-photo.jpg`] ] -- .right-column[ .line-height-1-5[<code> .</code> .muted[— Current directory]] .line-height-1-5[`..` .muted[— One level up from current directory]] ] ??? Demonstrates the difference between **absolute** and **relative** file paths. --- class: inverse center middle ## A Tour of <code>fs</code> --- ### Getting Common Directory Paths ```r > library(fs) > path_wd() ``` ``` /Users/garrick/work/core-r-course/slides ``` -- ```r > path_home() ``` ``` /Users/garrick ``` -- ```r > path_home_r() ``` ``` /Users/garrick/ ``` -- ```r > path_temp() ``` ``` /tmp/rsession-tempdir ``` --- ### Create a path ```r > path("data.csv") ``` ``` data.csv ``` -- ```r > path(path_home(), "data.csv") ``` ``` /Users/garrick/data.csv ``` -- ```r > path_rel(path(path_home(), "data.csv"), path_wd()) ``` ``` ../../../../garrick/data.csv ``` -- ```r > path(path_temp(), "data.csv") ``` ``` /tmp/rsession-tempdir/data.csv ``` --- ### Work with directories ```r > data_dir <- path(path_temp(), "data") > dir_exists(data_dir) ``` ``` /tmp/rsession-tempdir/data FALSE ``` -- ```r > dir_create(data_dir) ``` -- ```r > dir_ls(data_dir) ``` ``` character(0) ``` --- ### Download a file to the temp directory ```r > external_file <- "https://git.io/grk-hospital-referrals" > data_zip_file <- path(data_dir, "data.zip") > data_zip_file ``` ``` /tmp/rsession-tempdir/data/data.zip ``` -- ```r > download.file(external_file, destfile = data_zip_file) ``` ``` trying URL 'https://git.io/grk-hospital-referrals' Content type 'application/zip' length 79760 bytes (77 KB) ================================================== downloaded 77 KB ``` -- ```r > dir_ls(data_dir) ``` ``` /tmp/rsession-tempdir/data/data.zip ``` --- layout: true ### Extract the zip file **Option 1:** Use `file_show()` to open the directory and extract "manually". ```r file_show(data_dir) ``` **Option 2:** Use the `unzip()` function from .pkg[utils]. --- ```r ?unzip unzip(data_zip_file) # Unzips data into current working directory ``` ``` [1] "<wd>/ie-general-referrals-by-hospital/" [2] "<wd>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2015.csv" [3] "<wd>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2016.csv" [4] "<wd>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2017.csv" [5] "<wd>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2018.csv" [6] "<wd>/ie-general-referrals-by-hospital/README.txt" ``` --- ```r > unzip(data_zip_file, exdir = data_dir) ``` ``` [1] "<data_dir>/ie-general-referrals-by-hospital/" [2] "<data_dir>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2015.csv" [3] "<data_dir>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2016.csv" [4] "<data_dir>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2017.csv" [5] "<data_dir>/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2018.csv" [6] "<data_dir>/ie-general-referrals-by-hospital/README.txt" ``` --- layout: true ### List Files in A Directory with `dir_ls()` ```r > data_dir <- path(data_dir, "ie-general-referrals-by-hospital") ``` --- #### List the files inside a directory ```r > dir_ls(data_dir) ``` ``` /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/README.txt /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2015.csv /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2016.csv /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2017.csv /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2018.csv ``` --- #### Or using `path_rel()` to list the files relative to `data_dir` ```r > path_rel(dir_ls(data_dir), data_dir) ``` ``` README.txt general-referrals-by-hospital-department-2015.csv general-referrals-by-hospital-department-2016.csv general-referrals-by-hospital-department-2017.csv general-referrals-by-hospital-department-2018.csv ``` --- layout: true ### Get Info about Files in a Directory with `dir_info()` --- ```r > dir_info(data_dir) ``` ``` # A tibble: 5 x 18 # ... with 18 more variables: path <fs::path>, type <fct>, # size <fs::bytes>, permissions <fs::perms>, modification_time <dttm>, # user <chr>, group <chr>, device_id <dbl>, hard_links <dbl>, # special_device_id <dbl>, inode <dbl>, block_size <dbl>, blocks <dbl>, # flags <int>, generation <dbl>, access_time <dttm>, change_time <dttm>, # birth_time <dttm> ``` --- ```r > dir_info(data_dir) %>% + mutate(path = path_rel(path, data_dir)) ``` ``` # A tibble: 5 x 18 path type size permissions modification_time user group device_id hard_links special_device_… inode block_size blocks flags generation access_time change_time birth_time <fs::path> <fct> <fs::b> <fs::perms> <dttm> <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dttm> <dttm> <dttm> 1 README.txt file 184 rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 8 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 2 general-referrals-by-hospital-department-2015.csv file 57.4K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 120 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 3 general-referrals-by-hospital-department-2016.csv file 340.9K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 688 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 4 general-referrals-by-hospital-department-2017.csv file 241.4K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 488 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 5 general-referrals-by-hospital-department-2018.csv file 290.7K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 584 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 ``` --- ```r > dir_info(data_dir, regexp = "csv$") %>% + mutate(path = path_rel(path, data_dir)) ``` ``` # A tibble: 4 x 18 path type size permissions modification_time user group device_id hard_links special_device_… inode block_size blocks flags generation access_time change_time birth_time <fs::path> <fct> <fs::b> <fs::perms> <dttm> <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dttm> <dttm> <dttm> 1 general-referrals-by-hospital-department-2015.csv file 57.4K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 120 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 2 general-referrals-by-hospital-department-2016.csv file 340.9K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 688 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 3 general-referrals-by-hospital-department-2017.csv file 241.4K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 488 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 4 general-referrals-by-hospital-department-2018.csv file 290.7K rw-r--r-- 2018-09-25 09:58:04 4468… wheel 16777224 1 0 9.80e6 4096 584 0 0 2018-09-25 09:58:04 2018-09-25 09:58:04 2018-09-25 09:58:04 ``` --- layout: false ### Work with Files with `file_*()` ```r > csv_file <- dir_ls(data_dir, regexp = "csv$")[1] > file_exists(csv_file) %>% unname() # File Exists? ``` ``` [1] TRUE ``` -- ```r > file_access(csv_file) %>% unname() # File Accesible? ``` ``` [1] TRUE ``` -- ```r > file_copy(csv_file, path(path_wd(), "data_copy.csv")) # Copy File > dir_ls(regexp = "csv$") # Shows CSV files in working directory ``` ``` data_copy.csv ``` -- ```r > file_delete(path("data_copy.csv")) # Delete File ``` --- ### Work with _File Paths_ with `path_*()` ```r > path_file(csv_file) # File Name ``` ``` general-referrals-by-hospital-department-2015.csv ``` -- ```r > path_dir(csv_file) # File Directory ``` ``` /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital ``` -- ```r > path_ext(csv_file) # File Extension ``` ``` [1] "csv" ``` -- ```r > path_file(csv_file) %>% path_ext_remove() # Bare File Name ``` ``` general-referrals-by-hospital-department-2015 ``` --- layout: true ### Creating New Paths --- ```r > new_path <- path( + path_home(), + "Desktop", + path_ext_remove(path_file(csv_file)), + "data", + ext = path_ext(csv_file) + ) > new_path ``` ``` /Users/garrick/Desktop/general-referrals-by-hospital-department-2015/data.csv ``` --- ```r > new_path ``` ``` /Users/garrick/Desktop/general-referrals-by-hospital-department-2015/data.csv ``` -- #### Copy the `csv_file` to this new location: ```r file_copy(csv_file, new_path) ``` -- #### But then change your mind and delete the directory ```r dir_delete(csv_file, path_dir(new_path)) ``` --- layout: false class: center middle inverse # Why is this cool? -- ## Example:<br>Import All CSV Files From A Directory --- ### List our data files again ```r > data_dir <- path(path_temp(), "data", "ie-general-referrals-by-hospital") > csv_files <- dir_ls(data_dir, regexp = "csv$") > csv_files ``` ``` /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2015.csv /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2016.csv /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2017.csv /tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2018.csv ``` -- ```r > path_rel(csv_files, data_dir) ``` ``` general-referrals-by-hospital-department-2015.csv general-referrals-by-hospital-department-2016.csv general-referrals-by-hospital-department-2017.csv general-referrals-by-hospital-department-2018.csv ``` --- layout: true ### Read Using `readr` --- ```r library(readr) data <- list() for (csv_file in csv_files) { data[[csv_file]] <- read_csv(csv_file) } ``` ``` ## Parsed with column specification: ## cols( ## Month_Year = col_character(), ## Hospital_Name = col_character(), ## Hospital_ID = col_integer(), ## Hospital_Department = col_character(), ## ReferralType = col_character(), ## TotalReferrals = col_integer() ## ) ## Parsed with column specification: ## cols( ## Month_Year = col_character(), ## Hospital_Name = col_character(), ## Hospital_ID = col_integer(), ## Hospital_Department = col_character(), ## ReferralType = col_character(), ## TotalReferrals = col_integer() ## ) ## Parsed with column specification: ## cols( ## Month_Year = col_character(), ## Hospital_Name = col_character(), ## Hospital_ID = col_integer(), ## Hospital_Department = col_character(), ## ReferralType = col_character(), ## TotalReferrals = col_integer() ## ) ## Parsed with column specification: ## cols( ## Month_Year = col_character(), ## Hospital_Name = col_character(), ## Hospital_ID = col_integer(), ## Hospital_Department = col_character(), ## ReferralType = col_character(), ## TotalReferrals = col_integer() ## ) ``` --- ```r > names(data) ``` ``` [1] "/tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2015.csv" [2] "/tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2016.csv" [3] "/tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2017.csv" [4] "/tmp/rsession-tempdir/data/ie-general-referrals-by-hospital/general-referrals-by-hospital-department-2018.csv" ``` --- ```r library(readr) data <- list() for (csv_file in csv_files) { * data[[path_file(csv_file)]] <- read_csv(csv_file) } ``` ```r > names(data) ``` ``` [1] "general-referrals-by-hospital-department-2015.csv" [2] "general-referrals-by-hospital-department-2016.csv" [3] "general-referrals-by-hospital-department-2017.csv" [4] "general-referrals-by-hospital-department-2018.csv" ``` --- ```r > str(data, give.attr = FALSE) ``` ``` List of 4 $ general-referrals-by-hospital-department-2015.csv:Classes 'tbl_df', 'tbl' and 'data.frame': 837 obs. of 6 variables: ..$ Month_Year : chr [1:837] "Aug-15" "Aug-15" "Aug-15" "Aug-15" ... ..$ Hospital_Name : chr [1:837] "AMNCH" "AMNCH" "AMNCH" "Bantry General Hospital" ... ..$ Hospital_ID : int [1:837] 1049 1049 1049 704 704 704 704 704 704 704 ... ..$ Hospital_Department: chr [1:837] "Paediatric ENT" "Paediatric Gastroenterology" "Paediatric General Surgery" "Gastroenterology" ... ..$ ReferralType : chr [1:837] "General Referral" "General Referral" "General Referral" "General Referral" ... ..$ TotalReferrals : int [1:837] 2 4 4 12 18 43 8 9 5 5 ... $ general-referrals-by-hospital-department-2016.csv:Classes 'tbl_df', 'tbl' and 'data.frame': 4972 obs. of 6 variables: ..$ Month_Year : chr [1:4972] "Jan-16" "Jan-16" "Jan-16" "Jan-16" ... ..$ Hospital_Name : chr [1:4972] "AMNCH" "AMNCH" "AMNCH" "AMNCH" ... ..$ Hospital_ID : int [1:4972] 1049 1049 1049 1049 1049 1049 704 704 704 704 ... ..$ Hospital_Department: chr [1:4972] "Paediatric Cardiology" "Paediatric Dermatology" "Paediatric ENT" "Paediatric General Surgery" ... ..$ ReferralType : chr [1:4972] "General Referral" "General Referral" "General Referral" "General Referral" ... ..$ TotalReferrals : int [1:4972] 1 2 3 10 2 4 28 36 40 1 ... $ general-referrals-by-hospital-department-2017.csv:Classes 'tbl_df', 'tbl' and 'data.frame': 2977 obs. of 6 variables: ..$ Month_Year : chr [1:2977] "Jan-2017" "Jan-2017" "Jan-2017" "Jan-2017" ... ..$ Hospital_Name : chr [1:2977] "Cappagh National Orthopaedic Hospital" "Cavan Monaghan Hospital" "Cavan Monaghan Hospital" "Cavan Monaghan Hospital" ... ..$ Hospital_ID : int [1:2977] 955 402 402 402 402 402 402 402 402 402 ... ..$ Hospital_Department: chr [1:2977] "Orthopaedics" "Ante-Natal" "Dermatology" "ENT" ... ..$ ReferralType : chr [1:2977] "General Referral" "General Referral" "General Referral" "General Referral" ... ..$ TotalReferrals : int [1:2977] 19 9 27 39 34 2 4 33 32 125 ... $ general-referrals-by-hospital-department-2018.csv:Classes 'tbl_df', 'tbl' and 'data.frame': 3492 obs. of 6 variables: ..$ Month_Year : chr [1:3492] "Jan-2018" "Jan-2018" "Jan-2018" "Jan-2018" ... ..$ Hospital_Name : chr [1:3492] "Alliance Mater Private Cork Radiology" "Alliance Mater Private Cork Radiology" "Alliance Mater Private Cork Radiology" "Alliance Mater Private Cork Radiology" ... ..$ Hospital_ID : int [1:3492] 9116 9116 9116 9116 9116 9104 9104 9104 9104 9104 ... ..$ Hospital_Department: chr [1:3492] "Cardiac Calcium Scoring" "CT" "MRI" "Ultrasound" ... ..$ ReferralType : chr [1:3492] "General Referral" "General Referral" "General Referral" "General Referral" ... ..$ TotalReferrals : int [1:3492] 5 13 97 56 12 7 13 36 22 27 ... ``` --- layout: true ### Row-Bind Data Together --- Uses `bind_rows()` from .pkg[dplyr]: ```r > bind_rows(data) ``` ``` # A tibble: 12,278 x 6 Month_Year Hospital_Name Hospital_ID Hospital_Depart… ReferralType <chr> <chr> <int> <chr> <chr> 1 Aug-15 AMNCH 1049 Paediatric ENT General Ref… 2 Aug-15 AMNCH 1049 Paediatric Gast… General Ref… 3 Aug-15 AMNCH 1049 Paediatric Gene… General Ref… 4 Aug-15 Bantry Gener… 704 Gastroenterology General Ref… 5 Aug-15 Bantry Gener… 704 General Medicine General Ref… 6 Aug-15 Bantry Gener… 704 General Surgery General Ref… 7 Aug-15 Bantry Gener… 704 Medicine for th… General Ref… 8 Aug-15 Bantry Gener… 704 Outreach Dermat… General Ref… 9 Aug-15 Bantry Gener… 704 Outreach Orthop… General Ref… 10 Aug-15 Bantry Gener… 704 Outreach Surgic… General Ref… # ... with 12,268 more rows, and 1 more variable: TotalReferrals <int> ``` --- <table> <thead> <tr> <th style="text-align:left;"> Month_Year </th> <th style="text-align:left;"> Hospital_Name </th> <th style="text-align:right;"> Hospital_ID </th> <th style="text-align:left;"> Hospital_Department </th> <th style="text-align:left;"> ReferralType </th> <th style="text-align:right;"> TotalReferrals </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> Oct-16 </td> <td style="text-align:left;"> AMNCH </td> <td style="text-align:right;"> 1049 </td> <td style="text-align:left;"> Paediatric Developmental </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 3 </td> </tr> <tr> <td style="text-align:left;"> Dec-16 </td> <td style="text-align:left;"> UL Hospitals Group </td> <td style="text-align:right;"> 9100 </td> <td style="text-align:left;"> Nephrology </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 11 </td> </tr> <tr> <td style="text-align:left;"> Oct-2017 </td> <td style="text-align:left;"> Cork University Hospital </td> <td style="text-align:right;"> 724 </td> <td style="text-align:left;"> Neurology </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 33 </td> </tr> <tr> <td style="text-align:left;"> Feb-2018 </td> <td style="text-align:left;"> Midland Regional Hospital Portlaoise </td> <td style="text-align:right;"> 201 </td> <td style="text-align:left;"> Obstetrics </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 21 </td> </tr> <tr> <td style="text-align:left;"> May-2018 </td> <td style="text-align:left;"> St. Michael's Hospital </td> <td style="text-align:right;"> 912 </td> <td style="text-align:left;"> Ophthalmology </td> <td style="text-align:left;"> Ophthalmology Referral </td> <td style="text-align:right;"> 2 </td> </tr> </tbody> </table> --- ```r library(readr) *library(lubridate) data <- list() for (csv_file in csv_files) { data[[path_file(csv_file)]] <- read_csv(csv_file) } data <- bind_rows(data) %>% ``` --- ```r library(readr) library(lubridate) data <- list() for (csv_file in csv_files) { data[[path_file(csv_file)]] <- read_csv(csv_file) } data <- bind_rows(data) %>% * mutate(Month_Year = lubridate::myd(Month_Year, truncated = 1)) ``` -- Adding `truncated = 1` to `myd()` function lets it match on **Month** and **Year**. --- <table> <thead> <tr> <th style="text-align:left;"> Month_Year </th> <th style="text-align:left;"> Hospital_Name </th> <th style="text-align:right;"> Hospital_ID </th> <th style="text-align:left;"> Hospital_Department </th> <th style="text-align:left;"> ReferralType </th> <th style="text-align:right;"> TotalReferrals </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> 2016-10-01 </td> <td style="text-align:left;"> AMNCH </td> <td style="text-align:right;"> 1049 </td> <td style="text-align:left;"> Paediatric Developmental </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 3 </td> </tr> <tr> <td style="text-align:left;"> 2016-12-01 </td> <td style="text-align:left;"> UL Hospitals Group </td> <td style="text-align:right;"> 9100 </td> <td style="text-align:left;"> Nephrology </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 11 </td> </tr> <tr> <td style="text-align:left;"> 2017-10-01 </td> <td style="text-align:left;"> Cork University Hospital </td> <td style="text-align:right;"> 724 </td> <td style="text-align:left;"> Neurology </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 33 </td> </tr> <tr> <td style="text-align:left;"> 2018-02-01 </td> <td style="text-align:left;"> Midland Regional Hospital Portlaoise </td> <td style="text-align:right;"> 201 </td> <td style="text-align:left;"> Obstetrics </td> <td style="text-align:left;"> General Referral </td> <td style="text-align:right;"> 21 </td> </tr> <tr> <td style="text-align:left;"> 2018-05-01 </td> <td style="text-align:left;"> St. Michael's Hospital </td> <td style="text-align:right;"> 912 </td> <td style="text-align:left;"> Ophthalmology </td> <td style="text-align:left;"> Ophthalmology Referral </td> <td style="text-align:right;"> 2 </td> </tr> </tbody> </table> --- layout: false class: center top <img src="https://camo.githubusercontent.com/b7a8b1687a46868377f54dfc6288acd0bbd340e8/68747470733a2f2f692e696d6775722e636f6d2f4e4175783158632e706e67" width="50%"> ## Learn More at [`fs.r-lib.org`](https://fs.r-lib.org) Also [gerkelab.com/blog](https://www.gerkelab.com/blog/2018/09/import-directory-csv-purrr-readr/)